Paging in a GridView using an ObjectDataSource
You can get automatic paging in a GridView using an ObjectDataSource if you use it correctly. This example shows you how to combine it with Relatude.
The first thing you need to do is to to add a GridView and an ObjectDataSource control to your page:
<asp:GridView runat="server" ID="gridView2" AutoGenerateColumns="false" DataKeyNames="NodeId" AllowPaging="true" PageSize="20" DataSourceID="wafODS">
<Columns>
<asp:BoundField DataField="Name" HeaderText="Name" />
<asp:BoundField DataField="CreateDate" HeaderText="CreateDate" DataFormatString="{0:dd.MM.yy HH:mm}" />
</Columns>
</asp:GridView>
<asp:ObjectDataSource ID="wafODS" EnablePaging="true" runat="server" SelectCountMethod="GetArticlesCount" SelectMethod="GetArticles" TypeName="ArticleObjectDataSource"></asp:ObjectDataSource>
The ObjectDataSouce defines two methods, one SelectCountMethod and one SelectMethods. Those are the methods the ObjectDataSouce will call on the instance of the class it creates based on the TypeName property.
The GridView har set the DataSouceID property to the ID of the ObjectDataSource control. The methods on the ArticleObjectDataSource are implemented like this:
public class ArticleObjectDataSource
{
public List<ArticleBase> GetArticles(int startRowIndex, int maximumRows) {
int pageSize = maximumRows;
int pageIndex = 0;
int totalCount = 0;
if (startRowIndex > 0) {
pageIndex = (int)Math.Round(((double)startRowIndex / (double)pageSize));
}
return WAFContext.Session.Query<ArticleBase>().OrderBy(AqlArticleBase.PublishDate, true).Execute(pageIndex, pageSize, out totalCount);
}
public int GetArticlesCount() {
AqlQuery q = WAFContext.Session.CreateQuery();
AqlAliasArticleBase aliasArticleBase = new AqlAliasArticleBase();
AqlResultInteger count = q.Select(Aql.Count(aliasArticleBase.NodeId));
q.From(aliasArticleBase);
AqlResultSet rs = q.Execute();
int c = 0;
while (rs.Read()) {
c = count.Value;
}
return c;
}
}
The code is very simple, so I won't go into details, except for one gotcha. The GetArticles method got two parameters even though there are no parameters defined in the ObjectDataSource control. What's happening here is that the ObjectDataSource control have two properties called MaximumRowsParameterName and StartRowIndexParameterName. If those properties are defined in the control, the ObjectDataSource control passes the startRowIndex and maximunRows values to those parameters in the select method.
In our example, we haven't defined those properties, but they have two default parameter names, which are the same that we are using in the GetArticles method, which ensures that those values are passed to the method, enabling us to write code that only fetches the nodes for the current page.
The result:
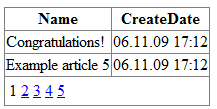